I found an issue with how the inventory is drawn that causes a crash when the size of the list the engine has to draws changes on specific conditions. From my testing, it can be triggered when consuming the last item of a stack while there are enough items in the inventory to require the list to scrol and the last item is visible on the last available position. If the last item is not visible or the inventory does not need to scroll because of the large amount of items, the crash won't trigger. The items themselves could have no use / icon / conditions whatsoever but so long as it is consumed and deleted, the crash will happen.
The traceback goes as follows:
WindowChoices.js:491 Uncaught TypeError: Cannot read property 'draw' of undefined at WindowChoices.draw (WindowChoices.js:491) at MenuInventory.drawHUD (MenuInventory.js:349) at Function.drawHUD (Stack.js:275) at loop (main.js:109) draw @WindowChoices.js:491 drawHUD @MenuInventory.js:349 drawHUD @Stack.js:275 loop @main.js:109 requestAnimationFrame (async) loop @main.js:97 requestAnimationFrame (async) // These last two lines repeat themselves a bunch of times after that Platform.js:147 TypeError: Cannot read property 'draw' of undefined at WindowChoices.draw (WindowChoices.js:491) at MenuInventory.drawHUD (MenuInventory.js:349) at Function.drawHUD (Stack.js:275) at loop (main.js:109) window.onerror @Platform.js:147
Looking in the files, the problem seems to be this loop here in the "draw()" function of "WindowChoices.js" (Line 491):
for (let i = 0; i < this.size; i++) { index = i + this.currentSelectedIndex - offset; this.listWindows[index].draw(true, this.listWindows[i].windowDimension, this.listWindows[i].contentDimension); // Here }
It complains of "this.listWindows[index]" being undefined and therefore having no ".draw()" property. I assume it has to do something with the draw still thinking the list option is there, maybe a problem with offset specifically. Im not sure however as I have little experience with JS and I haven't dug arround the code much.
If you want to reproduce my case specifically, fill an inventory tag with 23 consumable items (they can have no effect), scroll down to the last one and go back up 1, 2, 3, etc... As many as you want BUT making sure the last item in the list is still visible and being drawn. Then use one of the items (but not the last one) and the game should crash with the same error. This issue is a lot more common with the "All" filter as trying to use items near the end of the inventory will cause the crash. I tried on a different project, copy pasting default consumables (I used alarm clocks specifically) to fill the needed spaces and it crashes too so it isn't an issue with my custom icons, effects or conditions.
Any idea on how to solve/circunvent this that doesn't require either having less items or an inventory with a thousand filters to reduce list sizes?
If it is of any use locating the error, my system is a Windows 11 Home and im running RPG Paper Maker v.2.0.11.
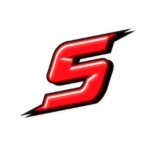
@wano (Sorry for the mention) I have been poking at it for a while and I think I came up with some sort of patch. It isn't perfect BUT it doesn't crash anymore and the inventory still works like it should from what im seeing. Esentially, I just added a boundary condition to ensure that "index" is within the valid range of this.listWindows to esentially skip those that are invalid or undefined. I just changed the loop in the code to look like this:
You might want to look into it regardless as im not very familiar with JS, nor the structure of the program either so there could be a better, more professional fix that I haven't found as this does not prevent invalid indexes to remain but skips them instead which could lead to other problems later that I haven't found yet. Still, for anyone with the same problem and until an offical patch is released, this can serve as a temporary fix. Just replace the old for loop with the one here and should be good to go for now.
I had a similar issue but it happened only with mouse use, is it happening only if using mouse or also with keyboard?